How to Build Your First AI Model Using Python
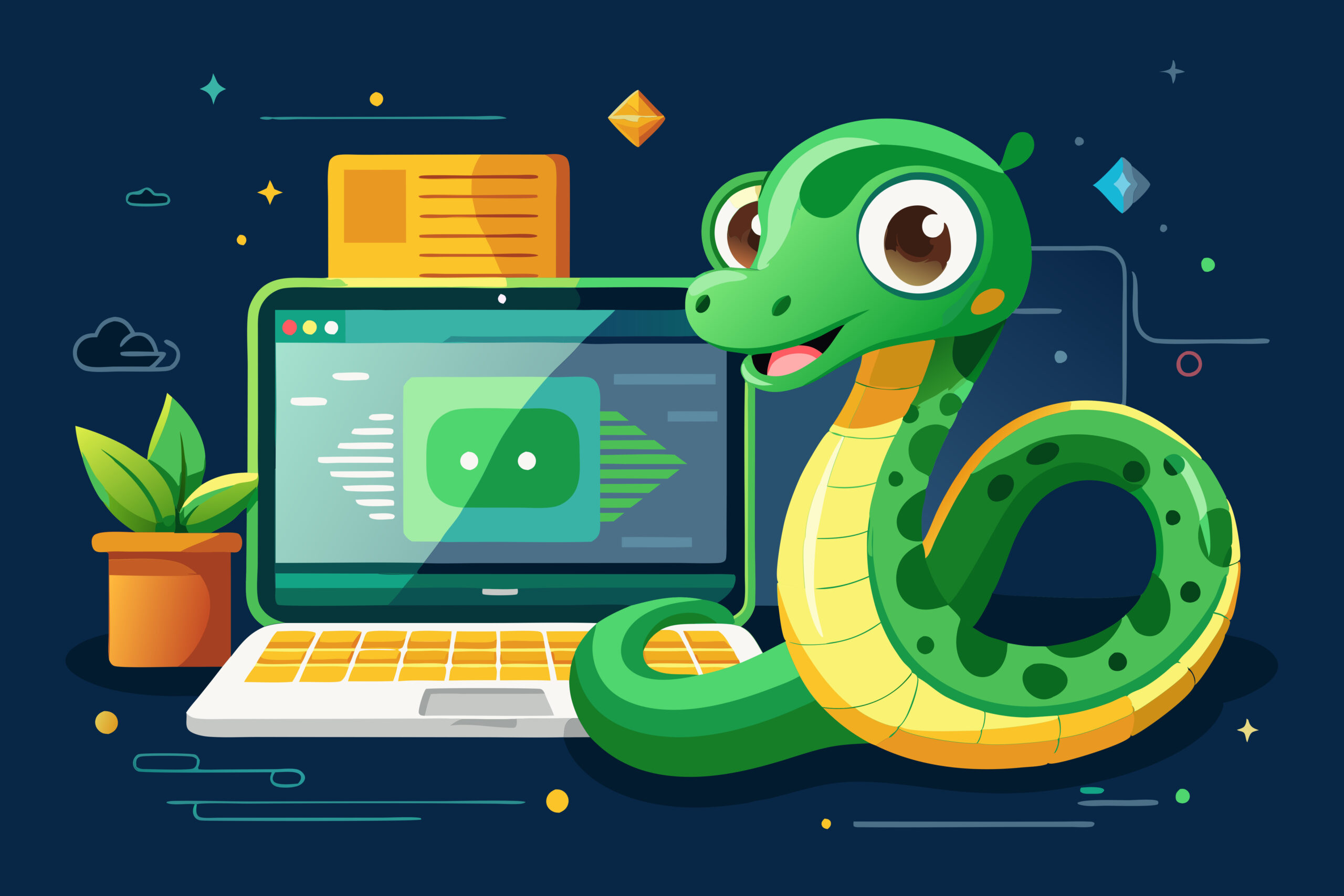
How to Build Your First AI Model Using Python
Artificial Intelligence (AI) is one of the most transformative technologies of our time, with applications ranging from natural language processing to self-driving cars. If you’re looking to break into AI and start building models, Python is the best programming language to use due to its ease of use, vast libraries, and active community. In this blog post, we’ll walk you through how to build your first AI model using Python. We’ll cover the essential technical concepts, provide a step-by-step guide, and highlight best practices, all with a focus on relevance in 2024.
Why Build an AI Model in Python?
Python has become the go-to language for AI and machine learning (ML) due to its simplicity and a broad range of libraries. These include popular frameworks like TensorFlow, PyTorch, Keras, and Scikit-learn, which allow developers to build sophisticated AI models quickly. With these libraries, you can build models for tasks such as image recognition, natural language understanding, and predictive analytics.
According to a 2023 Stack Overflow Developer Survey, Python remains one of the most popular languages among developers, particularly for AI and machine learning, making it the perfect starting point for your first AI model.
Step-by-Step Guide to Building Your First AI Model
Step 1: Set Up Your Environment
Before you start building your AI model, you’ll need to set up your development environment. This process involves installing Python and the necessary libraries for AI and machine learning development.
Tools You’ll Need:
- Python: Download the latest version of Python from the official Python website.
- Anaconda: Anaconda is a Python distribution that simplifies package management. You can download it here.
- Jupyter Notebook: A web-based IDE that allows you to write and run Python code interactively. This is included with Anaconda.
- Libraries: Install key Python libraries using pip (or conda):
pip install numpy pandas scikit-learn matplotlib
With these tools in place, you’re ready to start building.
Step 2: Understand the Basics of AI Models
An AI model is essentially a mathematical representation that learns patterns from data to make predictions. The process of building an AI model typically involves three main phases:
- Data Collection and Preparation
- Model Training
- Model Evaluation and Tuning
The quality of your data is crucial to the success of your AI model. For this guide, we will build a simple classification model using a popular dataset, the Iris dataset, which contains features of flowers used to classify them into different species.
Step 3: Load and Explore Your Dataset
To begin, we’ll load the Iris dataset using pandas, a library designed for data manipulation. Here’s how you can load and inspect the data:
import pandas as pd
from sklearn.datasets import load_iris
# Load the dataset
iris = load_iris()
# Convert to pandas DataFrame
df = pd.DataFrame(data=iris.data, columns=iris.feature_names)
df['species'] = iris.target
# Display the first few rows
print(df.head())
Step 4: Data Preprocessing
Before we can train our model, we need to preprocess the data. This involves splitting the dataset into features (inputs) and labels (outputs), as well as dividing the data into training and testing sets.
from sklearn.model_selection import train_test_split
# Features and labels
X = df.drop('species', axis=1)
y = df['species']
# Split data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
Step 5: Choose an Algorithm
For this tutorial, we’ll use a support vector machine (SVM), a popular algorithm for classification tasks. SVM works by finding the hyperplane that best separates the data into different classes.
from sklearn.svm import SVC
# Initialize the model
model = SVC()
# Train the model
model.fit(X_train, y_train)
Step 6: Evaluate Your Model
Once your model has been trained, you need to evaluate its performance on unseen data. We’ll use the test set for this purpose and calculate the accuracy score.
from sklearn.metrics import accuracy_score
# Make predictions on the test set
y_pred = model.predict(X_test)
# Calculate accuracy
accuracy = accuracy_score(y_test, y_pred)
print(f"Model Accuracy: {accuracy * 100:.2f}%")
Step 7: Tune Your Model (Optional)
Tuning your AI model involves adjusting hyperparameters to optimize its performance. For example, SVM has parameters like C
and kernel
that can be fine-tuned using grid search.
from sklearn.model_selection import GridSearchCV
# Define hyperparameter grid
param_grid = {'C': [0.1, 1, 10, 100], 'kernel': ['linear', 'rbf', 'poly']}
# Perform grid search
grid_search = GridSearchCV(SVC(), param_grid, refit=True, verbose=2)
grid_search.fit(X_train, y_train)
# Best parameters
print("Best Parameters:", grid_search.best_params_)
Step 8: Visualize Your Results
Visualization is essential for understanding your model’s performance. Python’s matplotlib
library can help you visualize the decision boundaries or the confusion matrix for classification.
import matplotlib.pyplot as plt
from sklearn.metrics import plot_confusion_matrix
# Confusion matrix
plot_confusion_matrix(model, X_test, y_test)
plt.title("Confusion Matrix")
plt.show()
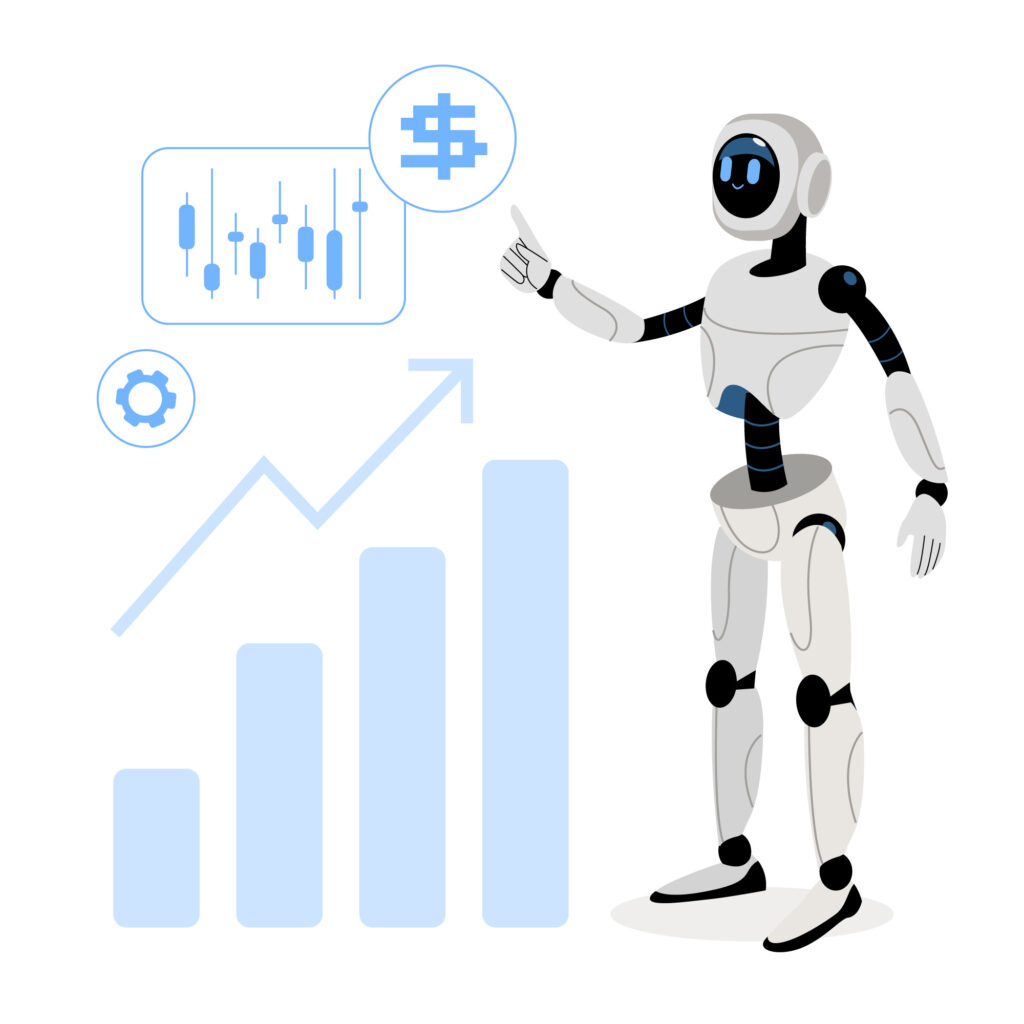
Practical Tips for Building AI Models
- Start with Simple Models: Don’t jump directly into complex neural networks. Begin with simpler models like decision trees, logistic regression, or SVM.
- Use Pre-built Datasets: When starting out, use well-known datasets such as Iris, MNIST, or Titanic. These are excellent for practice.
- Leverage Libraries: Python libraries like Scikit-learn, TensorFlow, and Keras simplify many aspects of AI development, from model creation to evaluation.
- Experiment: Try out different algorithms and compare their results. Experimentation is key to learning AI.
- Stay Updated: AI evolves quickly. Follow the latest research papers, attend AI webinars, and explore new libraries as they emerge.
The Future of AI in 2024
As of 2024, AI is continuing its rapid growth, and Python remains the dominant language for AI development. AI-driven automation, machine learning applications, and deep learning are driving innovation in industries such as healthcare, finance, and transportation. OpenAI’s models, such as GPT-4, and tools like Google AI are reshaping how businesses operate and how we interact with machines.
According to Gartner’s 2024 AI Impact report, AI adoption is projected to increase by over 30% in industries like banking and manufacturing. Therefore, learning how to build AI models will not only improve your skills but also position you as a valuable asset in a highly competitive job market.
Conclusion
Building your first AI model in Python is a rewarding experience that opens doors to a vast field of possibilities. By following the steps outlined in this guide, you now have the foundation to experiment with different algorithms, tune your models, and visualize your results. Remember, the key to mastering AI is consistent practice, experimentation, and staying up-to-date with new advancements.
Whether you are building a simple classification model or tackling complex deep learning problems, Python and its extensive ecosystem of libraries will be your best companion. As AI continues to evolve in 2024, now is the perfect time to start building your first AI model and contribute to the technology that is shaping the future.
—
With these tools and guidance, you are now ready to dive deeper into AI development. The possibilities are endless—start exploring today!